Stale Mocks
A Stale Mock is an object that was faked in a previous test and currently been used in another test. As a Stale Mock has no set behavior it could cause an unwanted behavior in your current test.
For Example:
C# public class ClassUnderTest { public static Dependency fooField ; public static int Foo() { return fooField.Bar(); } } public class Dependency { private int bar = 10; public int Bar() { return bar; } } [TestClass, Isolated] public class UnitTest1 { [TestMethod] public void CallingFakeInterfaceMethod_WillRerturn2() { //Arrange //setting the static field "fooField" as a fake of Dependency class var fakeDependency = Isolate.Fake.Instance<Dependency>(); ClassUnderTest.fooField = fakeDependency; Isolate.WhenCalled(() => ClassUnderTest.fooField.Bar()).WillReturn(2); //Act var res = ClassUnderTest.Foo(); //Assert Assert.AreEqual(2, res); } [TestMethod] public void CallingOriginalBarMethodWillReturn10() { //Act //When this test will run after the previous one the "fooField" will still be faked (a Stale Mock) //ClassUnderTest.Foo will return 0 as the "bar" field of Dependency hasn't been set to 10 becuase it was faked var res = ClassUnderTest.Foo(); //Assert Assert.AreEqual(10,res); } }
VB
Public Class ClassUnderTest
Public Shared fooField As Dependency
Public Shared Function Foo() As Integer
Return fooField.Bar()
End Function
End Class
Public Class Dependency
Private bar As Integer = 10
Public Function Bar() As Integer
Return bar
End Function
End Class
<TestClass,Isolated>
Public Class UnitTest1
<TestMethod>
Public Sub CallingFakeInterfaceMethod_WillRerturn2()
'Arrange
'setting the static field "fooField" as a fake of Dependency class
Dim fakeDependency = Isolate.Fake.Instance(Of Dependency)()
ClassUnderTest.fooField = fakeDependency
Isolate.WhenCalled(Function() ClassUnderTest.fooField.Bar()).WillReturn(2)
'Act
Dim res = ClassUnderTest.Foo()
'Assert
Assert.AreEqual(2, res)
End Sub
<TestMethod>
Public Sub CallingOriginalBarMethodWillReturn10()
'Act
'When this test will run after the previous one the "fooField" will still be faked (a Stale Mock)
'ClassUnderTest.Foo will return 0 as the "bar" field of Dependency hasn't been set to 10 becuase it was faked
Dim res = ClassUnderTest.Foo()
'Assert
Assert.AreEqual(10, res)
End Sub
End Class
Typemock will write Stale mock warnings to the output console.
A message will also appear on the Insight window:
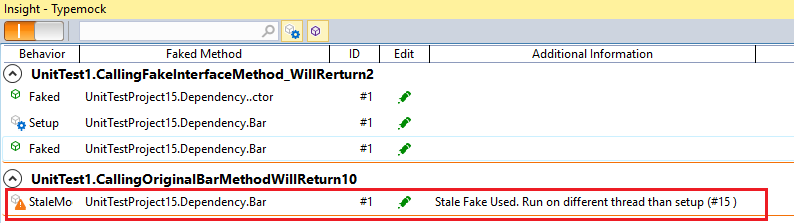
To ignore the warning message use the following syntax:
Syntax
C# [Isolated(IgnoreStaleMocks = true)]
VB
<Isolated(IgnoreStaleMocks:= true)>
This attribute can only be used on Class Level Attribute.