Modern C++ code often uses lambdas to express complex behavior in a concise and efficient way. However, lambdas can also introduce additional complexity and make unit testing more challenging. In particular, they can make it harder to isolate code for testing and to mock dependencies.
Let’s consider an example of how lambdas can make unit testing more challenging. Here’s a simple lambda function that filters the elements of a vector:
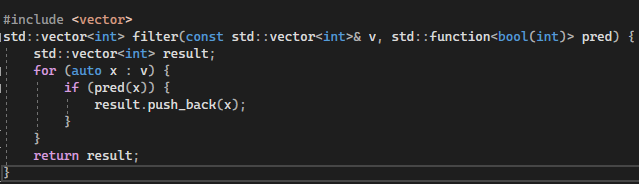
This code is simple and easy to understand, but it can be challenging to test. In particular, it relies on an external dependency, the std::function
class, and it uses a lambda function as an argument to the filter
function. To make this code testable, we need to find ways to isolate it from its dependencies and to test its behavior in isolation.
There are 2 things we can test. The first is that the lambda is used correctly. We can simply pass another lambda as an argument and test the code
Lambda used correctly
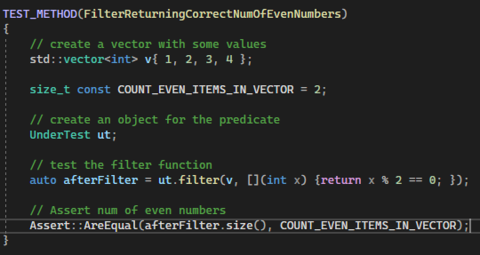
The second thing is to test the actual lambda. But the lambda is normally not visible.
Lambda used correctly
Typemock is a unit testing tool that allows us to create isolated test environments for our code. With Typemock, we can mock dependencies, intercept method calls, and simulate different scenarios to test our code in isolation. Here’s an example of how we could use Typemock to test the lambda
function:

Here is how we use Typemock to access the lambda so we can test it.
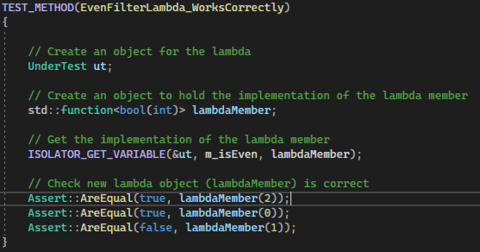
In this code, we use Typemock to access the lambda from the code and then it is easy to test.
This approach can simplify unit testing for code that uses lambdas and make it easier to write and maintain testable code.
In conclusion, modern C++ features such as lambdas can make code more expressive and efficient, but they can also introduce additional complexity and make unit testing more challenging. By using tools such as Typemock, we can simplify unit testing for modern C++ code that uses lambdas and write code that is easy to understand and is continuously tested