Unit Testing is Not Fun
Unit testing is like a trip to the dentist, it’s not always the most fun thing to do, but it’s necessary for a healthy codebase. But what if I told you that unit testing doesn’t have to be a painful experience? Introducing Google Test, the funnest framework for unit testing, and I’m not just saying that because it’s the only one I know.
Easy to Use
One of the best things about Google Test is that it’s like a choose-your-own-adventure book for unit testing. It’s easy to set up and use, even for developers who are new to unit testing. And with its well-documented library and plenty of resources available online, you’ll be laughing at those “unit testing is hard” jokes in no time
1 2 3 4 5 6 7 8 9 10 11 12 |
TEST_F(CalculatorTests, DivisionTest) { // Arrange int a = 15; int b = 5; // Act int result = calculator.Divide(a, b); // Assert ASSERT_EQ(result, 3); } |
In this example, we are using the Google Test framework to write unit tests for a class called “Calculator”
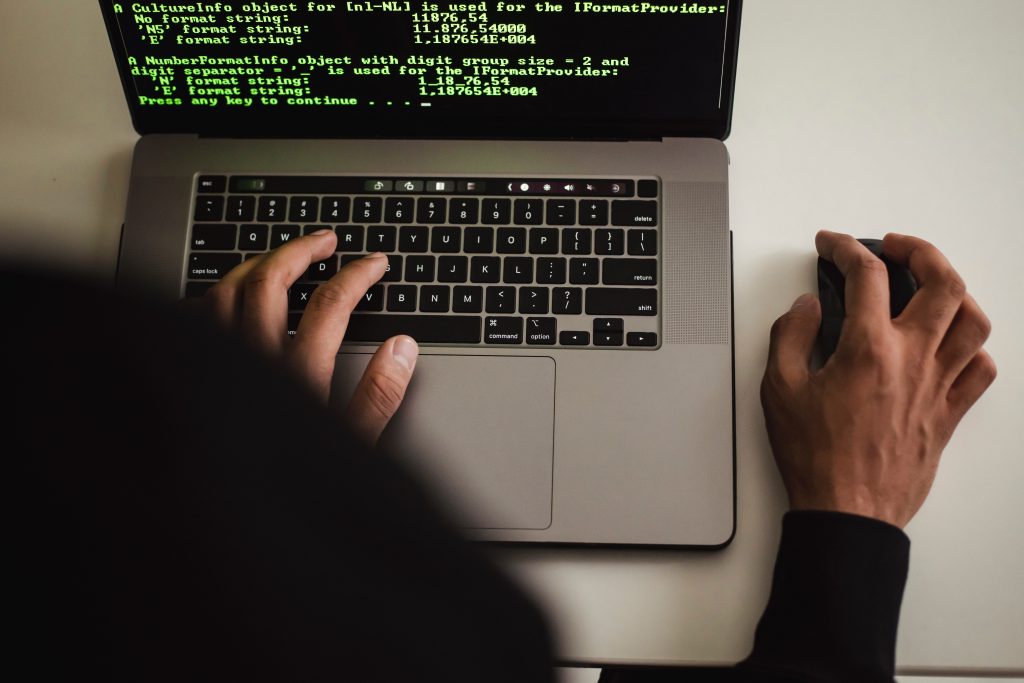
Flexible
Google Test is like the chameleon of frameworks, it can adapt to any project. It’s great for testing C++ and Java, and it can be used for both small and large codebases. Plus, it’s customizable, so you can make it your own and add your own personal flair
Want to learn more about unit testing with C/C++?
Powerful Features
Google Test is like the Swiss army knife of unit testing frameworks, it has everything you need. It includes support for test fixtures, test discovery, test filtering, and test execution. It also includes advanced features such as data-driven testing, so you can test the same code with different inputs and make sure it’s solid. And the built-in support for testing exceptions and memory leaks? It’s like having a personal code detective on call
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
TEST_F(CalculatorTests, DivisionByZeroTest) { // Arrange int a = 15; int b = 0; // Isolate the method call to prevent it from throwing an exception WHEN_CALLED(calculator.Divide(_,_)).Return(0); // Act int result = calculator.Divide(a, b); // Assert ASSERT_EQ(result, 0); } |
In this example, We are using TypeMock Isolator++ to isolate the method call to the “Divide” function and prevent it from throwing an exception when dividing by zero. (download!!!!)
Setting up Google Test – It’s easier then you think
The final countdown:
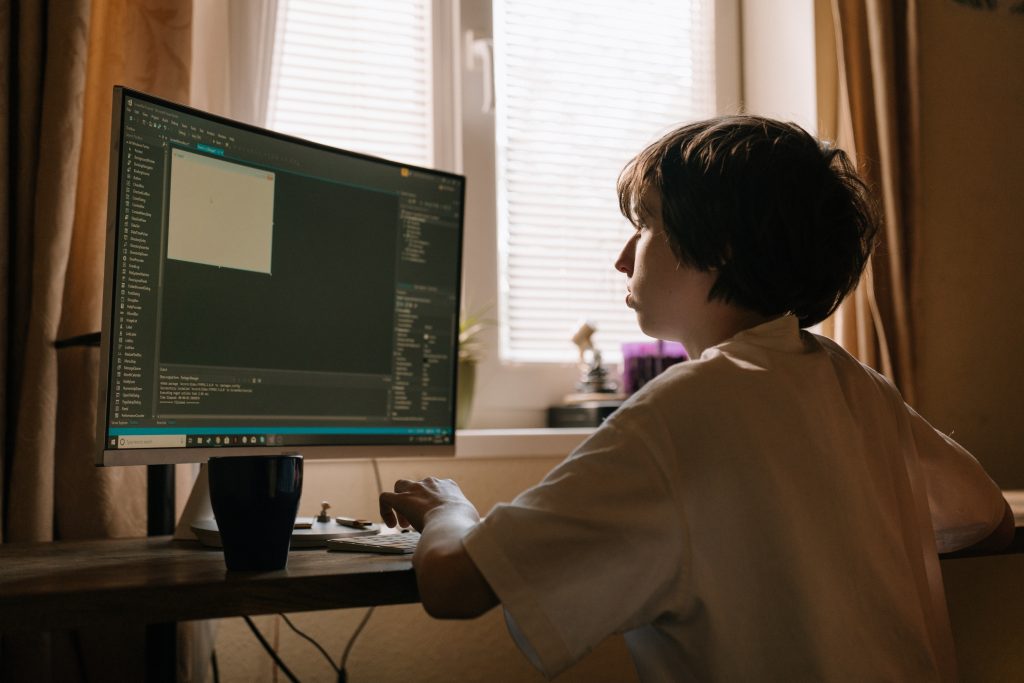
Google Test is like the perfect wingman for your unit testing needs. It’s easy to use, flexible, and packed with features. So, if you’re looking for a framework that can help you write better unit tests, Google Test is the one for you. With its simple syntax, powerful features, and wide range of support for different languages, it’s the perfect tool for any developer looking to make unit testing a fun experience